TypeScript Best Practices for 2025

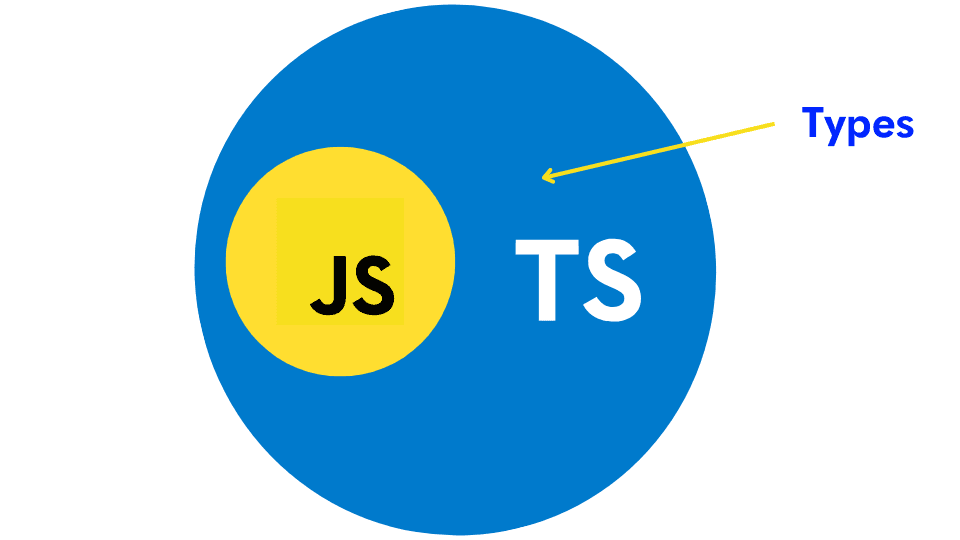
TypeScript Best Practices for 2025
TypeScript continues to evolve, and with it, our best practices. Here's what you need to know for 2024.
Type Safety First
Always aim for maximum type safety in your applications:
// Bad
function process(data: any) {
return data.value;
}
// Good
interface ProcessData {
value: string;
}
function process(data: ProcessData) {
return data.value;
}
Use Modern TypeScript Features
1. Template Literal Types
type IconSize = "sm" | "md" | "lg";
type IconVariant = "solid" | "outline";
type IconName = `icon-${IconSize}-${IconVariant}`;
2. Satisfies Operator
const theme = {
colors: {
primary: "#0099ff",
secondary: "#ff0099",
},
spacing: {
sm: "0.5rem",
md: "1rem",
},
} satisfies Record<string, Record<string, string>>;
Type Inference
Let TypeScript infer types when possible:
// Bad
const numbers: number[] = [1, 2, 3];
// Good
const numbers = [1, 2, 3];
Error Handling
Use discriminated unions for better error handling:
type Result<T> = { success: true; data: T } | { success: false; error: Error };
function processData<T>(result: Result<T>) {
if (result.success) {
return result.data;
} else {
console.error(result.error);
}
}
Conclusion
Following these practices will help you write more maintainable and safer TypeScript code.