Complete Guide to Next.js App Router

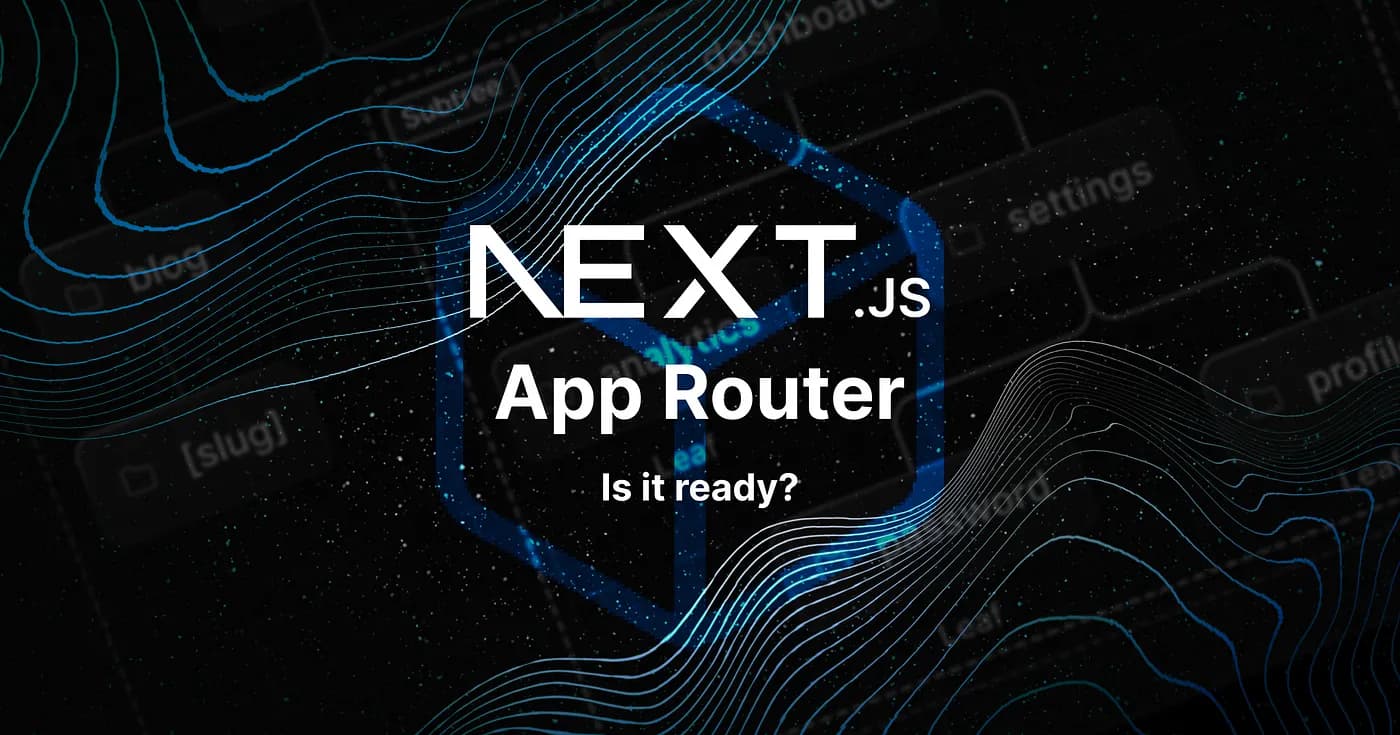
Complete Guide to Next.js App Router
Next.js App Router represents a paradigm shift in how we build React applications. This guide will help you understand the key concepts and best practices.
What is App Router?
The App Router is Next.js's new routing system built on React Server Components. It provides:
- First-class support for React Server Components
- Built-in data fetching
- Nested layouts and routes
- Improved performance through streaming
Key Features
1. Server Components
// app/page.tsx
export default async function Home() {
const data = await getData(); // This runs on the server!
return <h1>{data.title}</h1>;
}
2. Nested Layouts
// app/layout.tsx
export default function RootLayout({ children }) {
return (
<html>
<body>{children}</body>
</html>
);
}
3. Data Fetching
Next.js 13+ simplifies data fetching with built-in functions:
async function getData() {
const res = await fetch("https://api.example.com/data");
return res.json();
}
Best Practices
- Use Server Components by default
- Client Components when needed (interactivity)
- Colocate data fetching with components
- Leverage streaming for improved UX
Conclusion
The App Router is a powerful tool that helps build better React applications. Understanding these concepts will help you make the most of Next.js's capabilities.